最近DOTweenを使用したオブジェクト移動制御を実装する機会がありました。
かなり簡単かつ便利そうだったので紹介しようかと思います。
(さわりのさわりした紹介しません(できません))
DOTweenの導入
DOTweenのインポート
はじめにAssetStoreにてDOTweenを検索して、DOTweenをマイアセットに追加します。
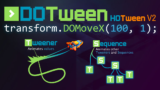
DOTween (HOTween v2) | アニメーション ツール | Unity Asset Store
Use the DOTween (HOTween v2) tool from Demigiant on your next project. Find this & more animation tools on the Unity Asset Store.
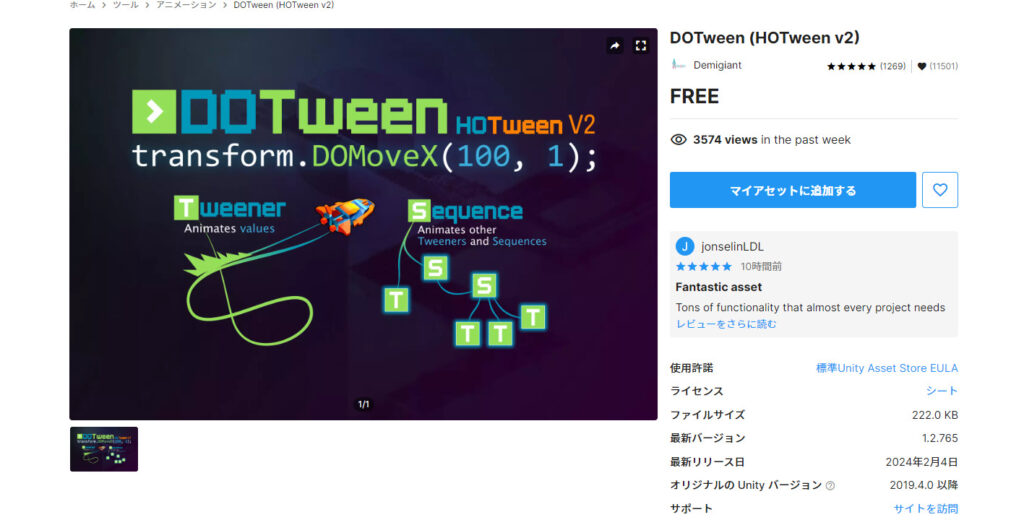
その後、UnityのPackageManagerからDOTweenをインポートします。
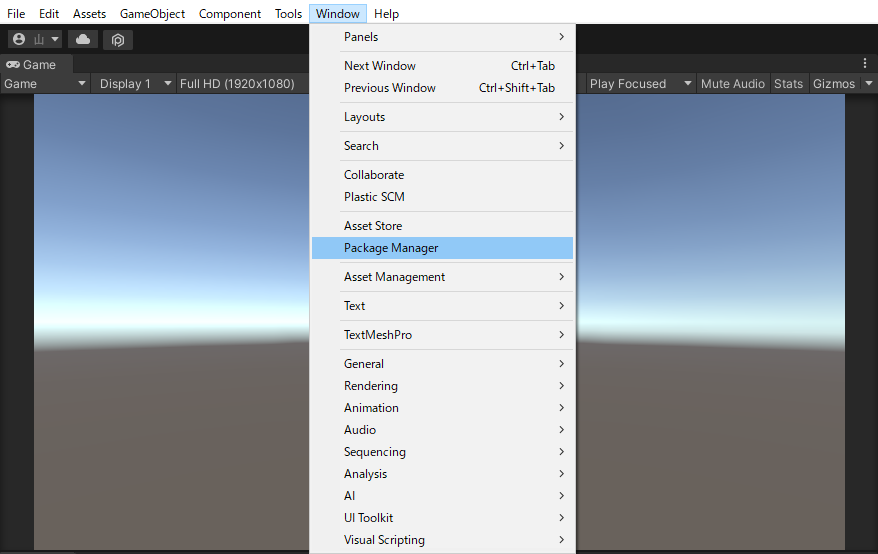
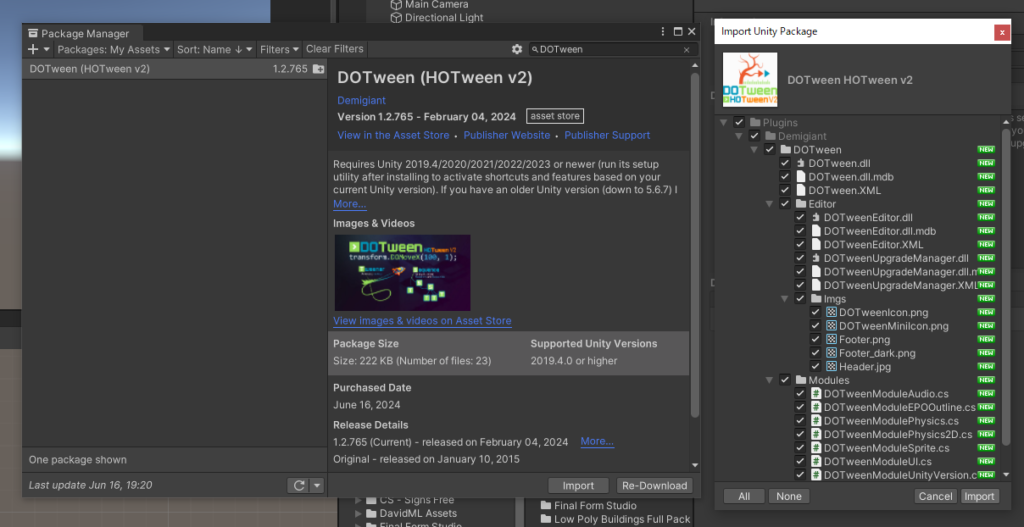
これでDOTweenのインポートは完了です。
DOTweenインポート後の初期設定
インポート後に表示される[New Version of DOTween Imported]ウィンドウの[Open DOTweeen Utility Panel]をクリックします。
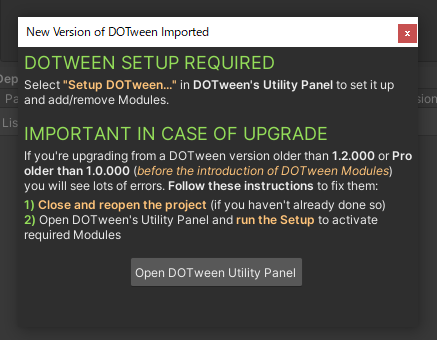
その後、[DOTween Utility Panel]ウィンドウの[Setup DOTween…]をクリックして[Apply]をクリックします。
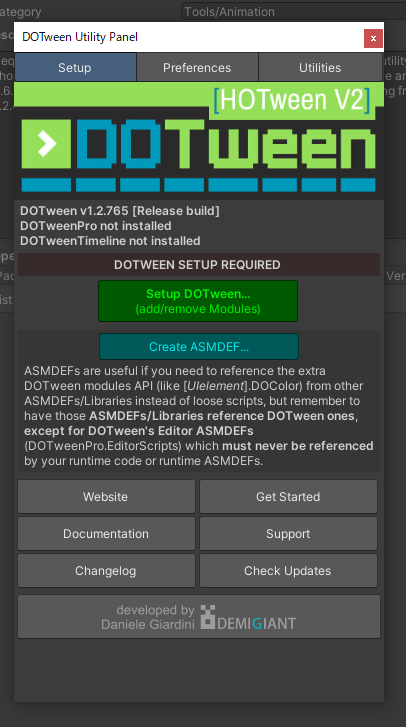
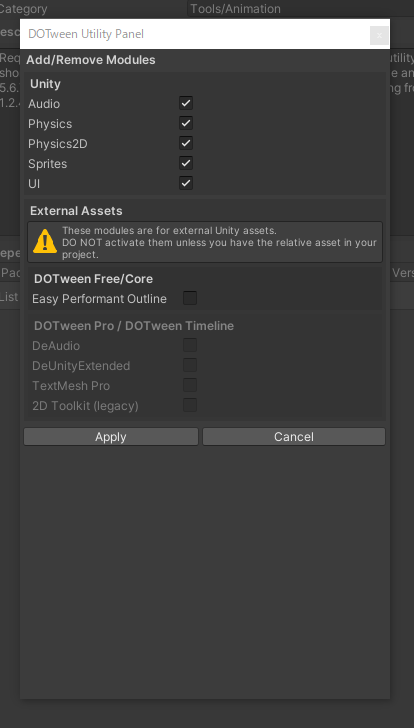
これでインポート後の初期設定は完了です。
DOTweenを使用したオブジェクト移動制御
簡単なオブジェクト移動制御方法を2パターン紹介します。
移動時間を設定したオブジェクト移動制御
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using DG.Tweening;//DOTween使用のため記載
public class DOTween_Sample : MonoBehaviour
{
[SerializeField,Tooltip("移動終了地点")]
private Transform m_goalPoint;
[SerializeField,Tooltip("移動時間")]
private float m_moveTime;
void Start()
{
//m_moveTimeで設定した時間でm_goalPointの位置まで移動する
this.transform.DOMove(m_goalPoint.position, m_moveTime).SetEase(Ease.Linear);
}
}
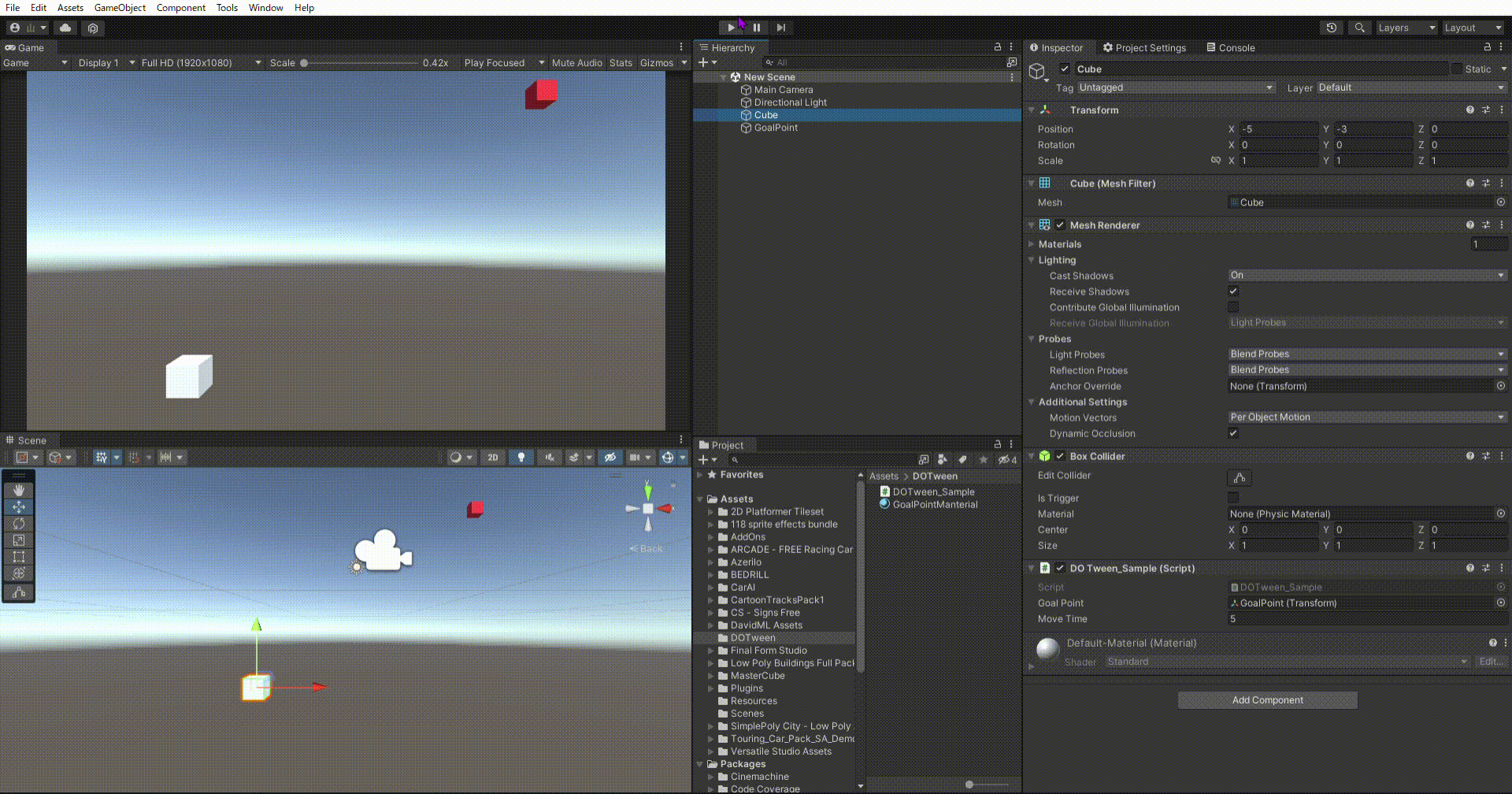
移動速度を設定したオブジェクト移動制御
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using DG.Tweening;//DOTween使用のため記載
public class DOTween_Sample : MonoBehaviour
{
[SerializeField,Tooltip("移動終了地点")]
private Transform m_goalPoint;
[SerializeField, Tooltip("移動スピード(s/m)")]
private float m_moveSpeed;
void Start()
{
//移動終了地点までの距離を取得
float distance = Vector3.Distance(this.transform.position, m_goalPoint.position);
//距離÷速さで移動時間を取得
float moveTime = distance/ m_moveSpeed;
//m_moveSpeedで設定したスピードでm_goalPointの位置まで移動する
this.transform.DOMove(m_goalPoint.position, moveTime).SetEase(Ease.Linear);
}
}
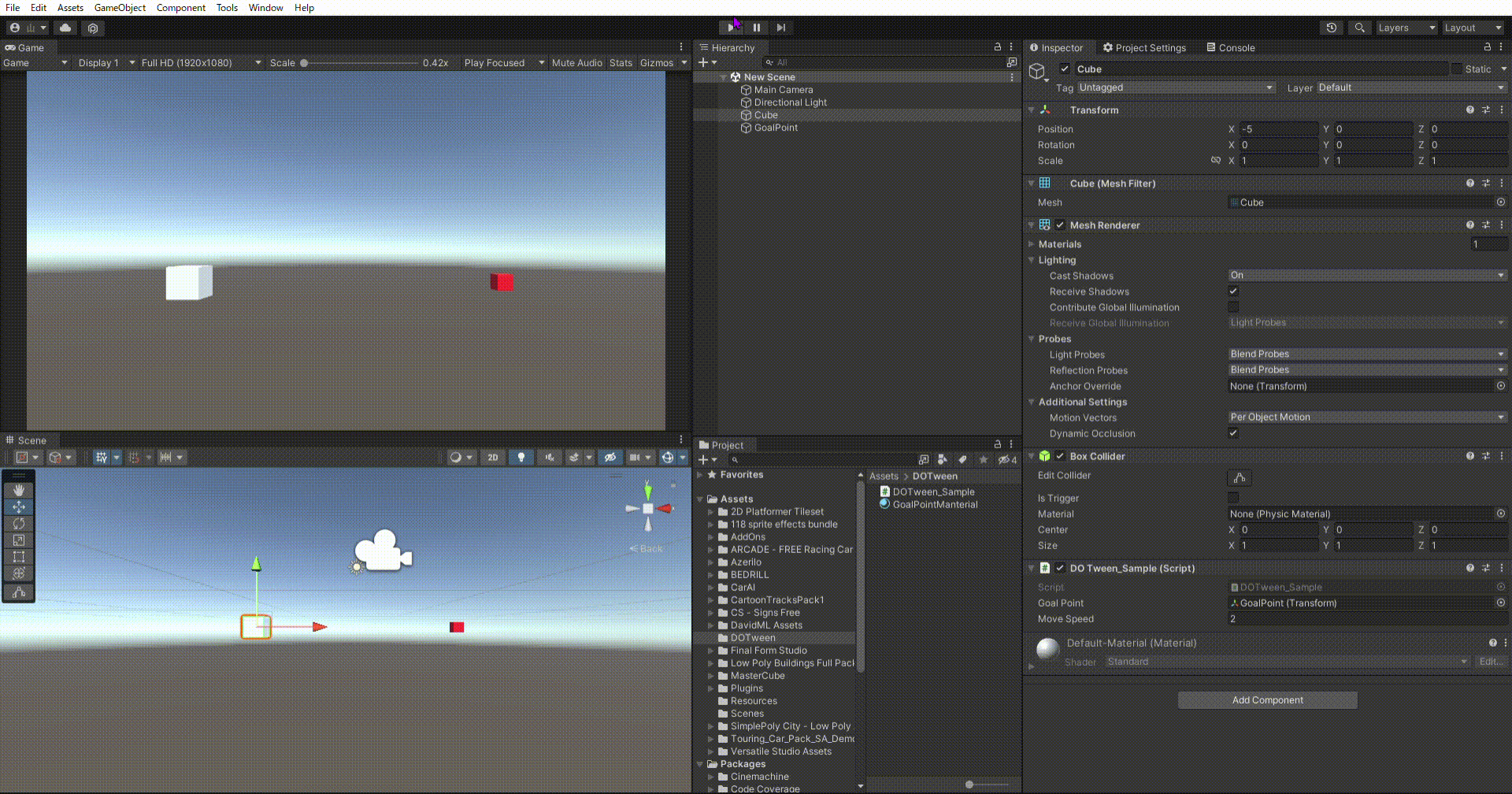
終わりに
今回は簡単な移動方法のみ紹介しましたが、複数の処理を組み合わせることで複雑な移動を実装することが出来ます。
時間があったら今後、詳しいところもまとめるかも?
コメント